Devlog #3 - Lobby System
I focused on building the foundation of the multiplayer experience by creating a lobby system. With the Master Server connection already in place from my previous work, I was able to move forward with creating a functional lobby. The lobby acts as a central hub where players can browse available rooms or choose to create a new one, becoming the host.
Lobby Structure
The lobby is designed to give players flexibility and control over how they start a game session. It presents two main options:
- Create Room
Players can create a new room with a customised name. This allows them to set up a private or public session based on their preferences, offering more control over who they play with.
- Join Room
Players can join public rooms by browsing the available list. Each active room is displayed as a button in the UI - clicking on one of these buttons will instantly join the corresponding room, making it quick and easy to find a match.
Creating a room
Create Room Menu UI showing room settings
CreateRoomMenu.cs
When a player decides to create a new room in the lobby, the OnCreateRoom() method is called. This function handles all the logic for setting up the room and sending a request to the Photon Master Server to actually create it.
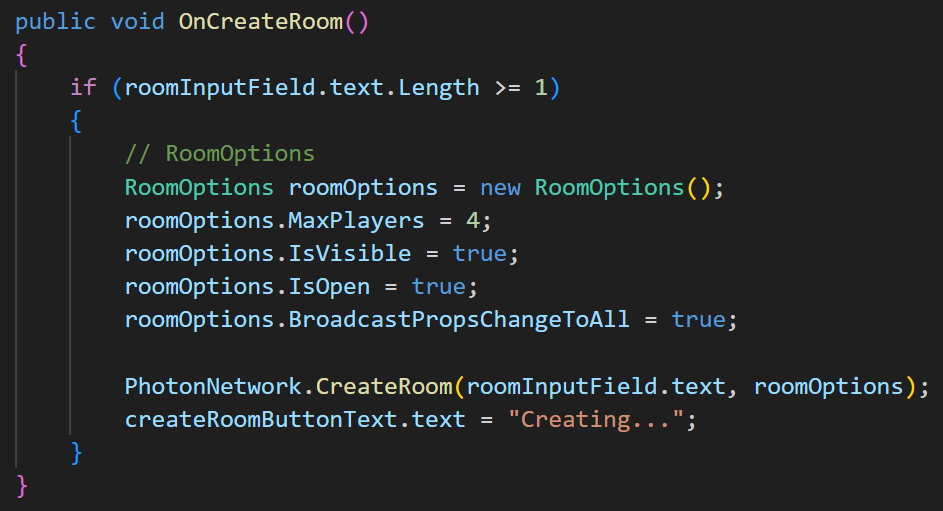
Setting Room Options
A new RoomOptions object is created to define how the room should behave. To get things started, I used some default settings for the room.
- MaxPlayer = 4: Limits the room to a maximum of 4 players.
- IsOpen = true: Allows other players to join the room after it's created.
- BroadcastPropsChangeToAll = true: Ensures that any updates to room or player properties are automatically broadcast to everyone in the room, handy for a ready-up status.
Creating the room
The core of this function is the call to PhotonNetwork.CreateRoom():
- The first argument is the room name provided by the player through the input field.
- The second argument is the RoomOptions object I just configured.
Updating the Room List
To make it easy for players to find available rooms, I developed a Find Room Menu that displays a list of all joinable rooms currently available on the server. The UI dynamically updates the list when new rooms have been created or removed.
Find Room Menu UI showing an active room
LobbyManager.cs
In this script, a Room Item prefab will be instantiated on the UI everytime a room has been created. It assigns the player count and name of the room.
- Instaniating Room Items
For every room in the roomList, a new UI object (RoomItem prefab) is instantiated inside the contentObject. This creates a dynamic list of rooms visible to the player.
- Setting Room Info
The room's name and current player count are assigned to the newly created RoomItem through two simple setup functions:
- SetUpRoomName(room.Name)
- SetUpPlayerCount(room.PlayerCount, room.MaxPlayers)
- Handling Full Rooms
Before displaying the room, the system checks if the room is already full:
- If the number of players is equal to or greater than MaxPlayers, the room item is displayed.
- If the room is still joinable, it stays visible.
- Tracking Room Items
Each instantiated RoomItem is added to a roomItemsList, allowing easy access later (e.g. refreshing or clearing the UI when the list updates).
Automatic Room List Updates
Photon automatically sends updates whenever rooms are created, joined or closed. To handle these updates efficiently, I override the OnRoomListUpdate method:
- Refresh Rate
To prevent updating the room list too frequently (which could cause performance issues), the room list only refreshes if enough time has passed (Time.time >= nextUpdateTime). This is controlled by a cooldown system using timeBetweenUpdates.
- Calling UpdateRoomList
When the refresh condition is met, it calls UpdateRoomList(roomList), which re-renders the room list UI based on the latest room data.
Joining a room
To allow players to easily connect to an existing room, I build a RoomItem system where each available room is represented by a clickable button in the lobby UI. When a player clicks on one of these room buttons, they automatically attempt to join that specific room.
JoinRoomMenu.cs and RoomItem.cs
Here's how the system works in code:
How It Works
RoomItem Script
- Each RoomItem prefab has the RoomItem.cs script attached to it. This script listens for a button click event:
- When a player clicks on the room button, the OnRoomItemSelect() method is called.
Passing the Room Name
- The OnRoomItemSelect() method grabs the text value (roomName.text) and passes it to the JoinRoom() function on the JoinRoomMenu.
Joining the Room
- JoinRoom(string roomName) calls PhotonNetwork.JoinRoom(roomName), which sends a request to the Photon server to join the room with the exact matching name.
- If the room exists and is not full, the player is connected directly to that room.
- If the room doesn't exist, Photon will automatically send a failure callback.
4 players joining a room
Milestones Met
The implementation of the Create Room Menu and Join Room Menu systems successfully met the expected outcomes and objectives set for this stage of development.
Room Creation
- Players can now create rooms with custom settings, including naming the room and setting the maximum number of players.
Dynamic Room List and Joining
- A working lobby system now displays available public rooms, and players can easily join by clicking on the room's button.
The room list updates efficiently without unnecessary refreshes, helping maintain good performance even when multiple rooms are active.
Next Steps...
After setting up the basic room creation and joining system, the next steps will focus on expanding and refining these features.
Planned improvements include:
Private / Public Settings
- Add more customisation options, by allowing the player to set a room to private or public. This gives them a chance to hide the room from the room list and be able to only share it with players they want to play a match with.
Join via Room Name
- Allow players to share a room name so their friends can easily join private rooms without searching through the room list.
Customisable Max Players
- Add more flexibility by letting players set the maximum number of players themselves when creating a room, rather than using a fixed value.
These updates will give players more control over how they play and connect with their friends, making the multiplayer experience more customisable.
References
[1] Photonengine. Setup and Connect (Version PUN 2). Available at: https://doc.photonengine.com/pun/current/getting-started/initial-setup.
[2] Photonengine. Introduction (Version PUN 2). Available at: https://doc.photonengine.com/pun/current/getting-started/pun-intro.
[3] Photonengine. Features Overview (Version PUN 2). Available at: https://doc.photonengine.com/pun/current/getting-started/feature-overview .
Dice & Domination
A high-stakes multiplayer strategy game with domination and combat
Status | In development |
Author | Shivani |
Genre | Strategy |
More posts
- Devlog #8 - Leaderboards with PlayFab51 days ago
- Devlog #7 - Upgrading the Lobby - From 2D to 3D55 days ago
- Devlog #6 - Character Customisation60 days ago
- Devlog #5 - User Authentication with PlayFab80 days ago
- Devlog #4 - Room Options94 days ago
- Devlog #2 - Multiplayer with PhotonFeb 27, 2025
- Devlog #1 - Programming ArchitectureFeb 10, 2025
Leave a comment
Log in with itch.io to leave a comment.